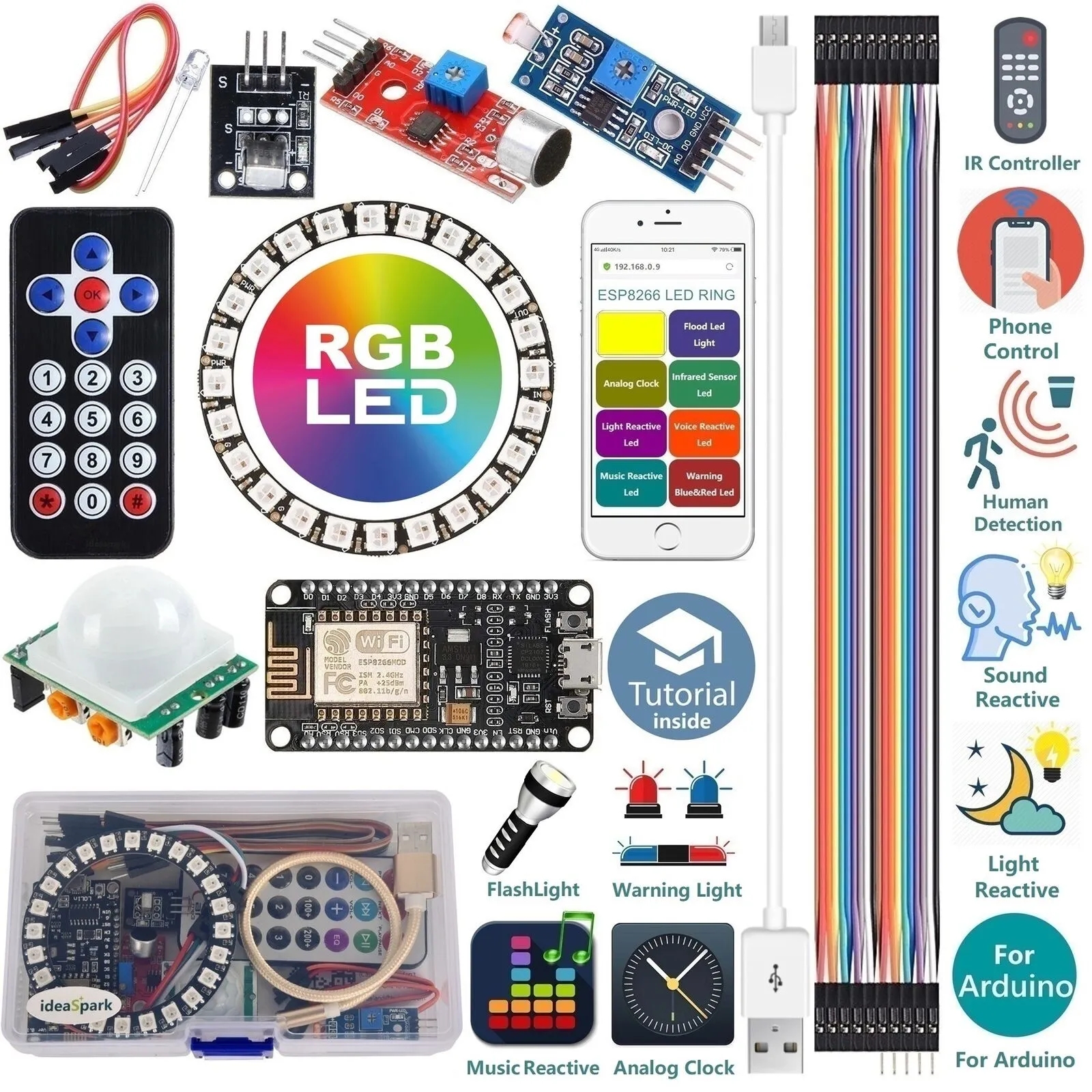
The world of electronics and programming can seem like a tangled web of wires and cryptic code. But fear not, aspiring inventors! Arduino, the open-source electronics platform, is here to cut through the confusion and empower anyone, regardless of skill level, to bring their creative visions to life. And the key to unlocking this world of possibility lies in the humble Arduino starter kit.
What’s in an Arduino Starter Kit?
Think of an Arduino starter kit as your personal electronics playground, packed with all the essential tools you need to get tinkering. These kits typically include:
- The Heart of it All: The Arduino Uno Board: This versatile microcontroller board is the brains behind your Arduino projects. It’s like a tiny computer that speaks the language of electronics, letting you control LEDs,motors, sensors, and much more.
- Jumper Wires: Your Circuit Connectors: These colorful cables are the handy messengers, carrying signals between your Arduino board and other components without the need for permanent soldering.
- Breadboard: Your Experimentation Platform: This reusable grid lets you build temporary circuits, making it easy to test and tweak your ideas without fear of frying anything.
- LEDs: Light Up Your Projects: These little light-emitting diodes come in a rainbow of colors, adding visual flair and feedback to your creations.
- Resistors: The Tiny Protectors: These unsung heroes keep your circuits safe by controlling the flow of electricity, preventing any unwanted smoke signals.
- Pushbuttons: Give Your Projects Some Control: These simple switches let you interact with your creations,adding a layer of fun and functionality.
- Potentiometers: Dial Up the Possibilities: These variable resistors act like tiny volume knobs, letting you fine-tune things like brightness, speed, or even the pitch of a buzzer.
- Sensors: See, Hear, and Feel the World: These amazing components act like the eyes and ears of your projects, detecting changes in temperature, light, motion, and more.
Why Choose an Arduino Starter Kit?
With an Arduino starter kit, you’re not just getting a box of parts; you’re getting a launchpad for your imagination. Here’s why it’s the perfect companion for your electronics adventure:
- Convenience: All the essentials are neatly gathered in one place, saving you time and money hunting for parts.
- Ease of Use: Most kits come with detailed instructions and tutorials, making it a breeze to get started, even with zero prior experience.
- Versatility: The possibilities are endless! From simple LED blinkers to mind-blowing robots, the components in your kit can be used to build a mind-boggling variety of projects.
- Learning Playground: Arduino is like STEM education in a fun and engaging package. Experimenting with these kits is a fantastic way to grasp the fundamentals of electronics and programming, all while having a blast.
Best Arduino Starter Kits for Eager Beginners:
Choosing the best Arduino starter kit can feel like picking your favorite candy in a treasure trove. But worry not, I’ve handpicked a few top contenders for you:
- Official Arduino Starter Kit: This kit, straight from the Arduino family, is a fantastic all-rounder. It includes everything you need to get going, plus a project book with 15 step-by-step tutorials to fuel your creativity.
- Elegoo Uno R3 Project Kit: This kit is perfect for aspiring roboticists. It comes with an Uno R3 board, servo motors, and other goodies you need to build a basic robot arm and conquer the world (or at least your desk).
- Freenove Ultimate UNO R3 Starter Kit: This comprehensive kit is like an electronics buffet, offering everything you need to build a smorgasbord of projects, from basic circuits to complex robots and home automation systems. It’s the ultimate playground for the curious mind.
Unleash Your Creativity with Arduino Starter Kit Projects:
Now that you have your Arduino starter kit in hand, it’s time to unleash your inner mad scientist! Here are a few project ideas to get your creative juices flowing:
Project Ideas for Beginners
Here come the fun part and hands-on learning! If you’re new to Arduino, it’s best to start with simple projects that teach you the basics. Here are some exciting ideas you can explore:
LED Blink
This is a classic Arduino project that teaches you how to control an LED using a digital output pin.
const int ledPin = 8;
void setup() {
pinMode(ledPin, OUTPUT);
}
void loop() {
digitalWrite(ledPin, HIGH); // turn the LED on
delay(1000); // wait for one second
digitalWrite(ledPin, LOW); // turn the LED off
delay(1000); // wait for one second
}
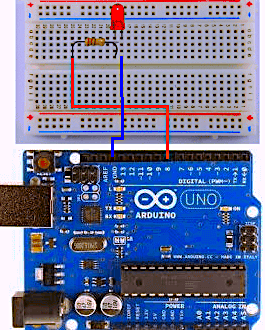
Button
This project teaches you how to read the state of a pushbutton and use it to control an LED.
const int buttonPin = 2;
const int ledPin = 3;
void setup() {
pinMode(buttonPin, INPUT);
pinMode(ledPin, OUTPUT);
}
void loop() {
if (digitalRead(buttonPin) == HIGH) {
digitalWrite(ledPin, HIGH);
} else {
digitalWrite(ledPin, LOW);
}
}
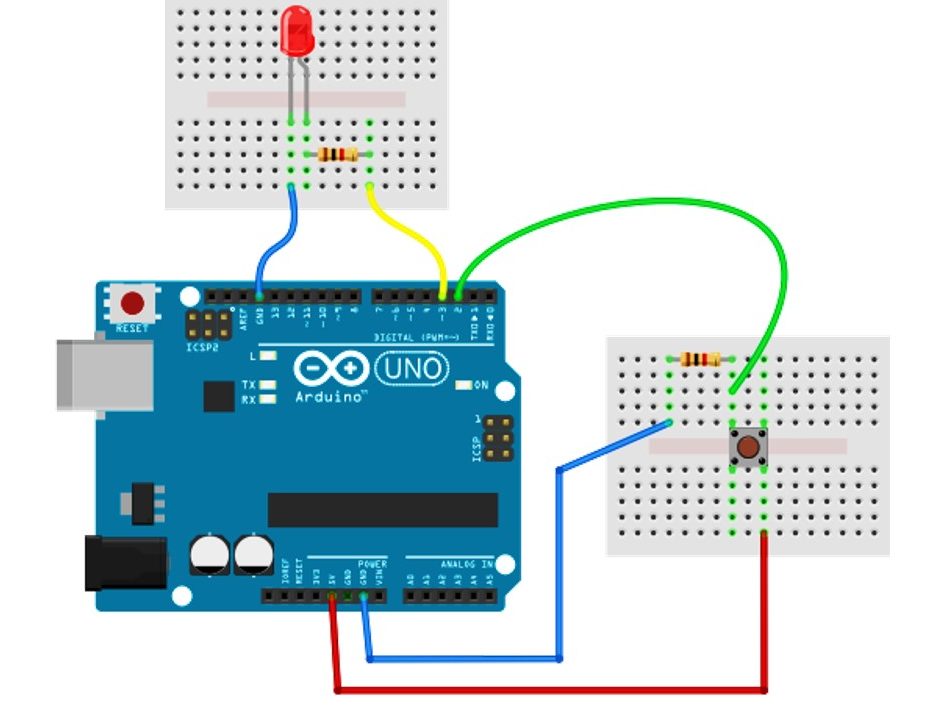
Potentiometer
This project teaches you how to use a potentiometer to control the brightness of an LED.
const int potPin = A1;
const int ledPin = 11;
void setup() {
pinMode(ledPin, OUTPUT);
}
void loop() {
int potValue = analogRead(potPin);
int ledBrightness = map(potValue, 0, 1023, 0, 255);
analogWrite(ledPin, ledBrightness);
}
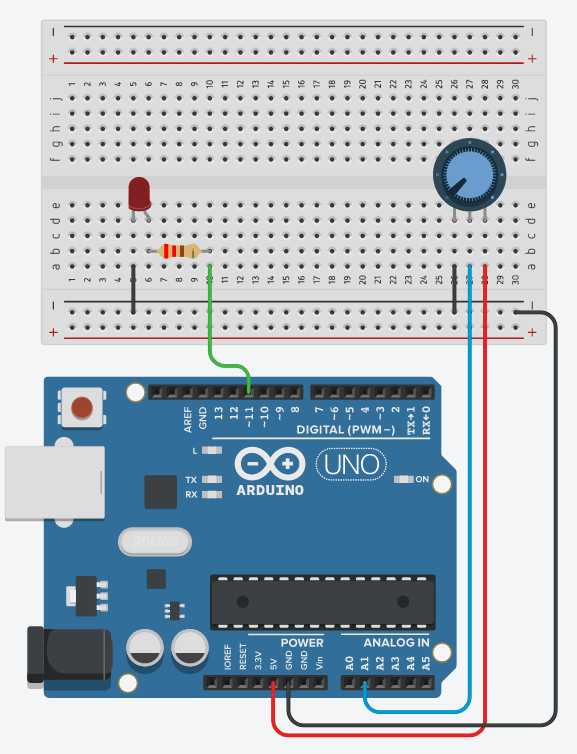
Buzzer
This project teaches you how to make an Arduino buzzer make a sound using a PWM output pin.
const int buzzerPin = 9;
void setup() {
pinMode(buzzerPin, OUTPUT);
}
void loop() {
tone(buzzerPin, 1000);
delay(500);
noTone(buzzerPin);
delay(500);
}
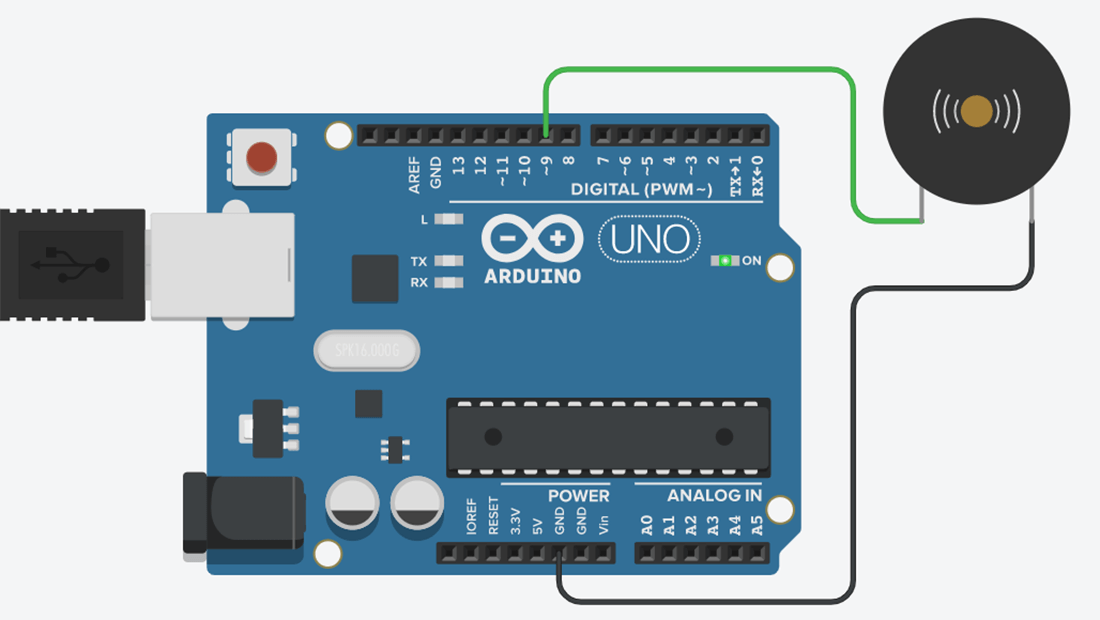
Temperature Sensor
This project teaches you how to read the temperature from a sensor and if it goes above a certain value, play a buzzer.
const int sensorPin = A0;
const int buzzerPin = 13;
void setup() {
pinMode(buzzerPin, OUTPUT);
}
void loop() {
int sensorValue = analogRead(sensorPin);
float temperature = sensorValue * 0.0048828125;
if (temperature > 25) {
digitalWrite(buzzerPin, HIGH);
} else {
digitalWrite(buzzerPin, LOW);
}
delay(1000);
}
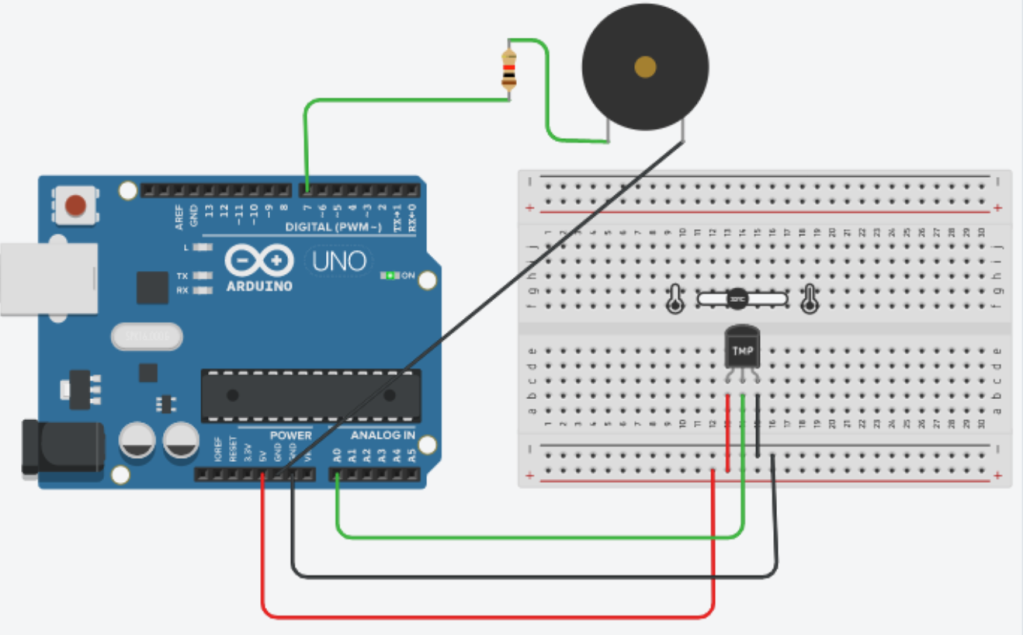
Light Sensor
This project teaches you how to read the light level from a sensor and use it to control an LED.
const int sensorPin = A0;
const int ledPin = 3;
void setup() {
pinMode(ledPin, OUTPUT);
}
void loop() {
int sensorValue = analogRead(sensorPin);
int lightLevel = map(sensorValue, 0, 1023, 0, 100);
if (lightLevel < 50) {
digitalWrite(ledPin, HIGH);
} else {
digitalWrite(ledPin, LOW);
}
delay(1000);
}
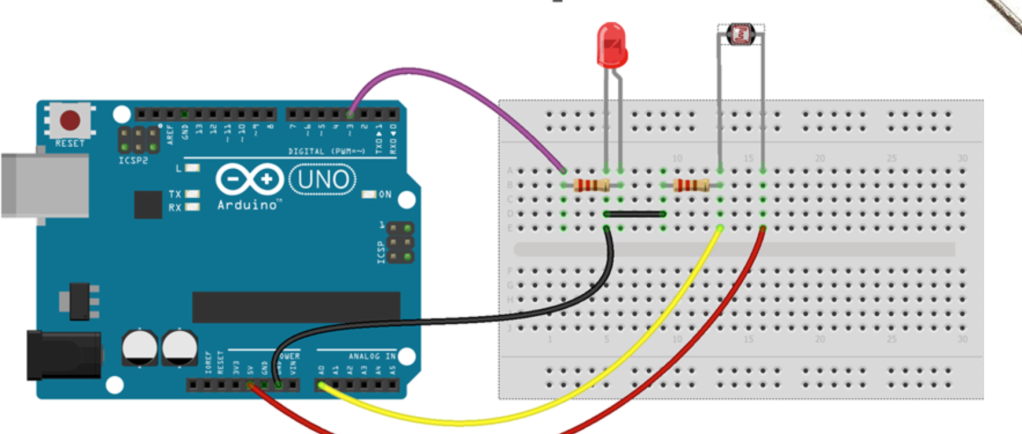
Intermediate and Advanced Projects
As you gain confidence and experience with Arduino, you can explore more complex projects. These go beyond the basics and provide a more in-depth learning experience. We’ve outlined a few of these projects below, and you can start with them once you’ve mastered the beginner projects. Let’s move on to the next level:
Servo Motor
This project teaches you how to control a servo motor using a PWM output pin.
#include <Servo.h>
Servo myservo;
void setup() {
myservo.attach(9);
}
void loop() {
myservo.write(0);
delay(1500);
myservo.write(90);
delay(1500);
}
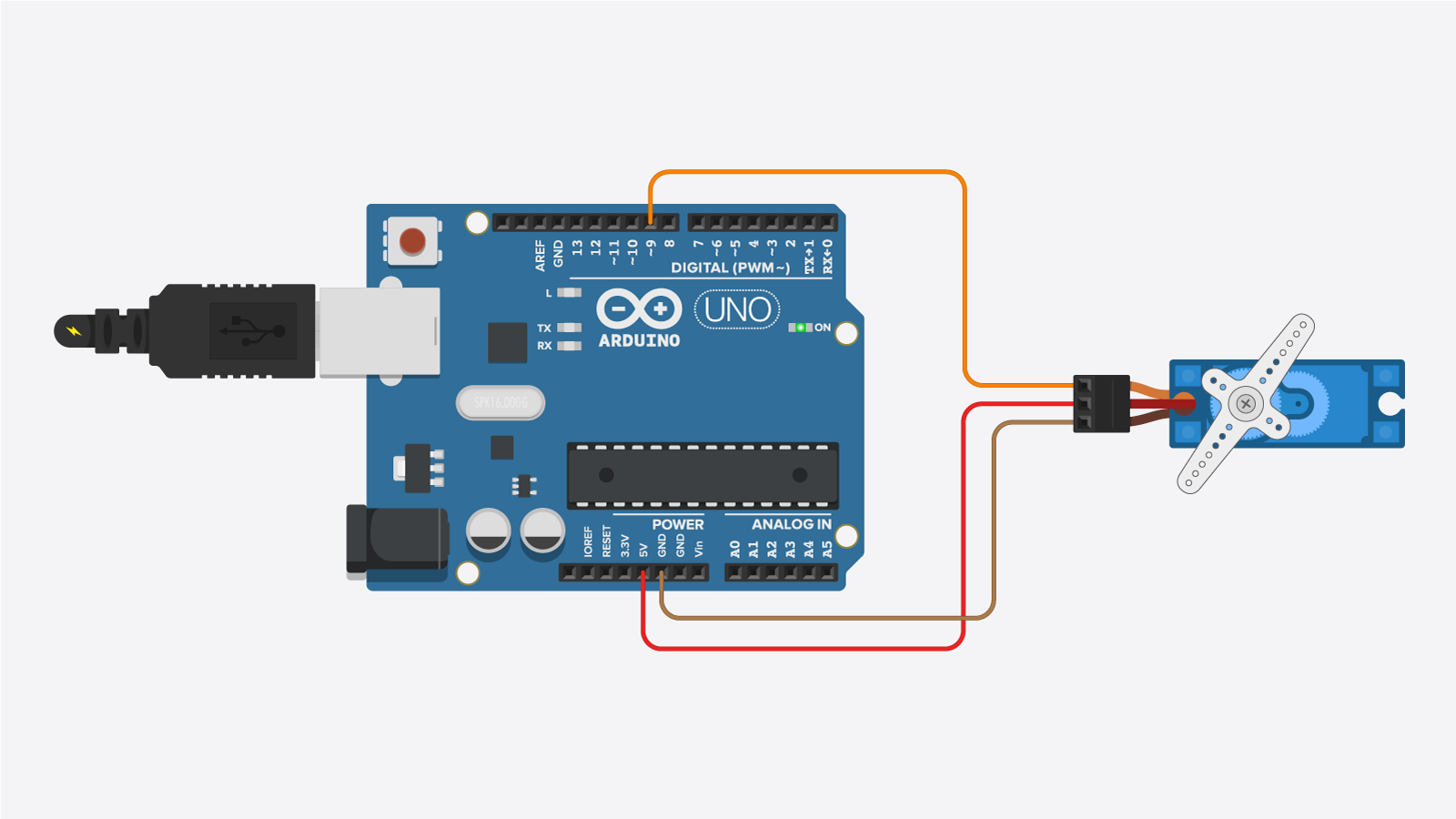
DC Motor
This project teaches you how to control a DC motor using an Arduino motor shield.
#include <ArduinoMotorShield.h>
ArduinoMotorShield amsh(3);
void setup() {
amsh.begin();
amsh.setSpeed(AMotor::M1, 255);
}
void loop() {
amsh.run(AMotor::M1, FORWARD);
delay(5000);
amsh.run(AMotor::M1, REVERSE);
delay(5000);
}
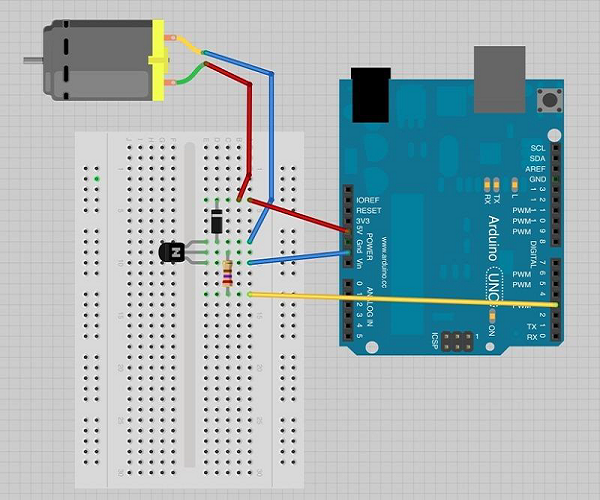
Bluetooth Communication
This project teaches you how to use a Bluetooth module to communicate with an Arduino.
#include <SoftwareSerial.h>
SoftwareSerial bluetoothSerial(2, 3);
void setup() {
bluetoothSerial.begin(9600);
}
void loop() {
if (bluetoothSerial.available()) {
String incomingMessage = bluetoothSerial.readString();
Serial.println(incomingMessage);
if (incomingMessage == "ON") {
digitalWrite(13, HIGH);
} else if (incomingMessage == "OFF") {
digitalWrite(13, LOW);
}
}
}
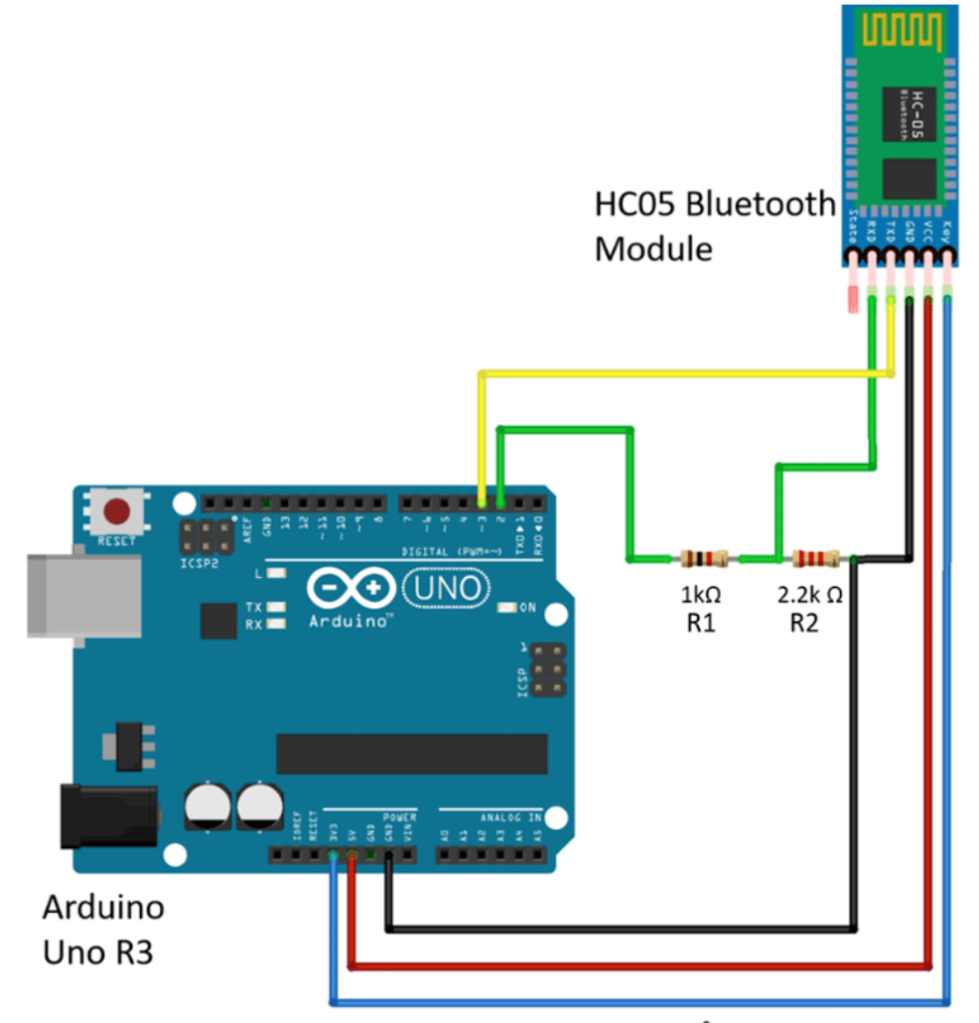
There are lot of Android and ISO mobile apps to communicate with this bluetooth modules and you can use one of those app to connect and send messages to the module.
Conclusion
In this article, we’ve embarked on a journey through the world of Arduino, from its fundamentals to exciting project ideas. With the right starter kit and a dash of creativity, everyone including children can explore the limitless possibilities of DIY electronics. So, dust off your Arduino starter kit, dive into these projects, and let your imagination run wild. After all, Arduino is not just a microcontroller board; it’s a path to endless innovation. It’s time to bring your ideas to life.
FAQ
- What if I’m new to programming and electronics? Is Arduino suitable for beginners?
- Absolutely! Arduino is designed to be beginner-friendly. Many tutorials and resources cater to newcomers. You can start with simple projects and gradually build your skills.
- Where can I find tutorials and guidance for Arduino projects?
- You’ll find many Arduino tutorials and guides online. Websites like Arduino.cc, Instructables, and YouTube channels offer step-by-step instructions and video demonstrations.
- What should I do if I encounter problems or errors in my projects?
- Don’t be discouraged by errors; they are part of the learning process. Arduino has a supportive community on forums like Arduino Stack Exchange and Reddit. Describe your issue, and fellow enthusiasts will help you troubleshoot.
- Can I use an Arduino kit for educational purposes or STEM projects?
- Yes, Arduino is a fantastic tool for STEM education. Many schools and educators use it to teach electronics and programming. The hands-on experience it provides is invaluable for students.
- How can I join the Arduino community and connect with like-minded enthusiasts?
- You can connect with the Arduino community through various online forums, social media groups, and local maker spaces. Participate in discussions, share your projects, and learn from others in the community.